Create and publish a NuGet package using Visual Studio
- Visual Studio 2022 Installation:
- To begin, you’ll need to install Visual Studio 2022 for Windows. This can be done by downloading the free Community edition from visualstudio.microsoft.com. Alternatively, if you possess licenses, you can opt for the Professional or Enterprise edition.
- NuGet Capabilities:
- Visual Studio 2017 and later versions automatically incorporate NuGet capabilities upon installing any .NET-related workload. This simplifies package management within your projects.
- .NET CLI Installation (if necessary):
- If the .NET CLI (Command Line Interface) isn’t already installed on your system, it’s essential to do so. For Visual Studio 2017 and newer versions, the .NET CLI comes bundled with any .NET Core-related workload installation. However, if you’re using a different setup or require standalone installation, you’ll need to install the .NET Core SDK. The .NET CLI is particularly crucial for projects utilizing the SDK-style format, indicated by the SDK attribute. Notably, the default .NET class library template in Visual Studio 2017 and later incorporates the SDK attribute.
Registration and Installation
Before you can leverage NuGet’s package management capabilities and effectively contribute to the .NET ecosystem, it’s imperative to complete a few initial steps to register and install the necessary tools. This preface outlines the essential actions required to ensure seamless integration with NuGet.
- NuGet.org Account Registration:
- If you haven’t already, you’ll need to register for a free account on nuget.org. This account is pivotal for managing and uploading NuGet packages. Upon registration, be sure to confirm your account to unlock its full functionality.
- NuGet CLI Installation:
- To interact with NuGet from the command line, you’ll need to install the NuGet CLI. This can be accomplished by downloading the nuget.exe file from nuget.org. Once downloaded, place the nuget.exe file in a suitable directory and ensure that this directory is added to your system’s PATH environment variable. This enables seamless access to the NuGet CLI from any command prompt or terminal session.
By completing these preliminary steps, you’ll be equipped with the necessary credentials and tools to seamlessly integrate NuGet into your development workflow. Let’s proceed with these setup tasks to ensure a smooth transition into utilizing NuGet for package management within your .NET projects.
Creating a Class Library Project
Before you embark on the journey of packaging your code into a NuGet package, you’ll need to establish a foundation by creating a class library project. This preface provides step-by-step guidance on setting up your project within Visual Studio.
- Launch Visual Studio and Begin Project Creation:
- Open Visual Studio and navigate to the menu bar. From there, select File > New > Project to initiate the project creation process.
- Specify Project Type and Template:
- In the “Create a new project” window, ensure that you’ve selected C# as the programming language, Windows as the platform, and Library as the project type from the dropdown lists.
- Choose Class Library Template:
- From the list of project templates, locate and select Class Library. This template is designed for creating a class library that targets either .NET or .NET Standard. Proceed by selecting Next.
- Configure Project Details:
- In the “Configure your new project” window, designate a meaningful name for your project, such as calculator. Then, select Next to proceed.
- Select Framework and Create Project:
- In the “Additional information” window, choose an appropriate framework for your project. If uncertain, opting for the latest framework version is a prudent choice, as it can be adjusted later if needed. Once you’ve made your selection, proceed by selecting Create.
- Verify Project Creation:
- To ensure the successful creation of your project, navigate to Build > Build Solution. Confirm that the project builds without errors. You can locate the resulting DLL within the Debug folder (or Release if that configuration was used).
- Optional: Add Functional Code (if desired):
- While not necessary for this quickstart, you have the option to include additional code within your class library project. If you opt to do so, you can add the provided functional code to enhance the functionality of your NuGet package.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace Calc
{
public class Calculator
{
public int Add(int x, int y)
{
return x+y;
}
}
}
Configuring Package Properties
Once your project is established, the next crucial step is configuring the properties of your NuGet package. This preface provides a comprehensive guide on customizing the settings of your package within Visual Studio.
- Navigate to Project Properties:
- Begin by selecting your project within the Solution Explorer. Then, navigate to Project > [Your Project Name] Properties. Replace [Your Project Name] with the actual name of your project.
- Access Package Settings:
- Within the project properties window, locate and expand the Package node. From there, select General to access the package properties.
- Note on SDK-style Projects:
- It’s important to note that the Package node only appears for SDK-style projects in Visual Studio. If your project is targeting a non-SDK style (typically .NET Framework), you have two options:
- Migrate the project to an SDK-style project to access the package settings within Visual Studio.
- Refer to alternative resources such as “Create and publish a .NET Framework package” for step-by-step instructions tailored to non-SDK style projects.
- It’s important to note that the Package node only appears for SDK-style projects in Visual Studio. If your project is targeting a non-SDK style (typically .NET Framework), you have two options:
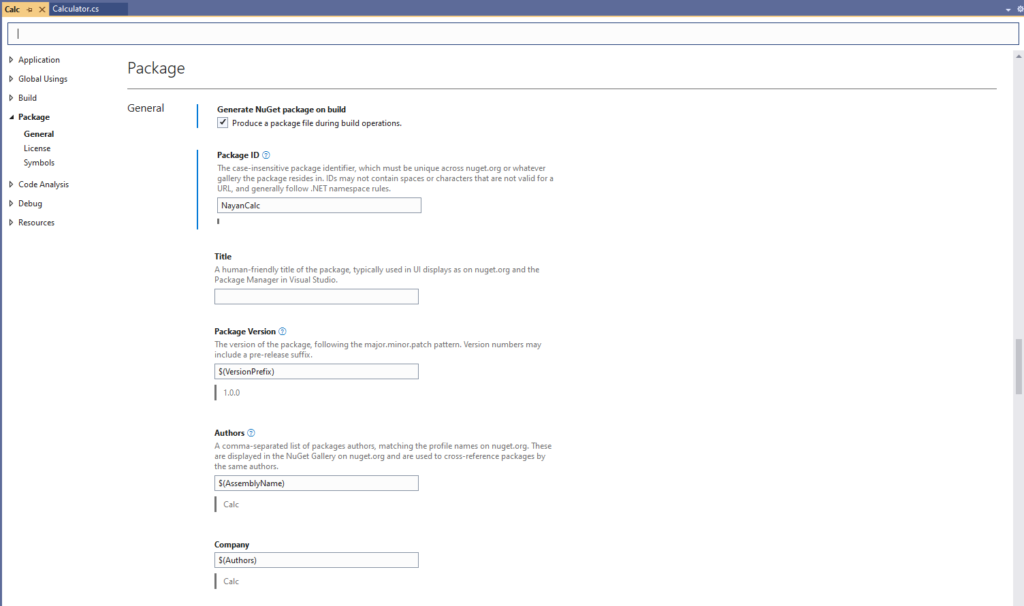
Give your package a unique Package ID and fill out any other desired properties. For a table that shows how MSBuild properties (SDK-style projects) map to .nuspec file properties, see pack targets. For a description of .nuspec file properties, see the .nuspec file reference. All of these properties go into the .nuspec
manifest that Visual Studio creates for the project.
Running the Pack Command
- Set Active Solution Configuration:
- Begin by navigating to Build > Configuration Manager within Visual Studio. From there, ensure that the active solution configuration is set to Release. This configuration ensures that the generated NuGet package is optimized for distribution.
- Select Project and Pack:
- Next, locate and select your project (in this case, the “Calculator ” project) within the Solution Explorer. With the project selected, choose the Pack option. This action prompts Visual Studio to initiate the packaging process.
- Package Creation:
- Visual Studio will then proceed to build your project and create the corresponding NuGet package. Upon completion, the .nupkg file, containing your packaged project, will be generated.
- Examine Output Details:
- To confirm the successful creation of the NuGet package, examine the Output window within Visual Studio. This window provides detailed information about the build process, including the path to the generated package file. Typically, the path will be within the “bin\Release\net8.0” directory, corresponding to a .NET 8.0 target.
Publish the package
Acquiring Your API Key
Prior to publishing your NuGet package, it’s essential to create an API key to authenticate the publishing process. This preface outlines the steps required to generate and acquire your API key from nuget.org.
- Sign into nuget.org:
- Begin by signing into your nuget.org account. If you don’t have an account already, you’ll need to create one to proceed.
- Navigate to API Keys:
- After signing in, locate your user name at the upper right corner of the screen. Click on your user name, and then select API Keys from the dropdown menu.
- Create API Key:
- Within the API Keys section, select Create. You’ll be prompted to provide a name for your key to help identify its purpose.
- Select Push Scope:
- Under Select Scopes, ensure that Push is selected. This scope grants the necessary permissions for pushing packages to nuget.org.
- Define Package Glob Pattern:
- In the Select Packages > Glob Pattern field, enter an asterisk (*) to allow pushing packages with any name.
- Generate API Key:
- Once all necessary configurations are in place, select Create to generate your API key.
- Copy API Key:
- After the key is created, select Copy to copy the newly generated API key to your clipboard. This key will be used to authenticate the publishing process when pushing your NuGet package.
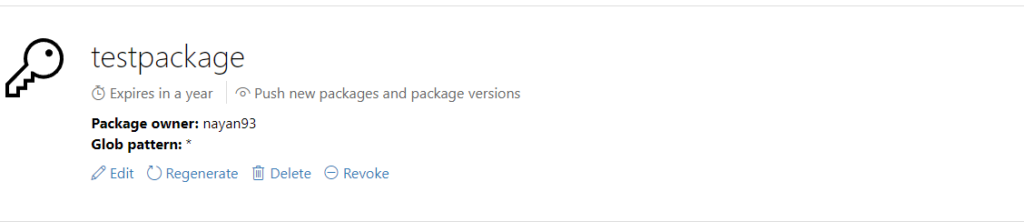
Publish with the .NET CLI or NuGet CLI
Each of the following CLI tools allows you to push a package to the server and publish it. Select the tab for your CLI tool, either .NET CLI or NuGet CLI.
Using the .NET CLI (dotnet.exe) is the recommended alternative to using the NuGet CLI.
From the folder that contains the .nupkg file, run the following command. Specify your .nupkg filename, and replace the key value with your API key.
dotnet nuget push Contoso.08.28.22.001.Test.1.0.0.nupkg --api-key qz2jga8pl3dvn2akksyquwcs9ygggg4exypy3bhxy6w6x6 --source https://api.nuget.org/v3/index
Use NuGet package in other project
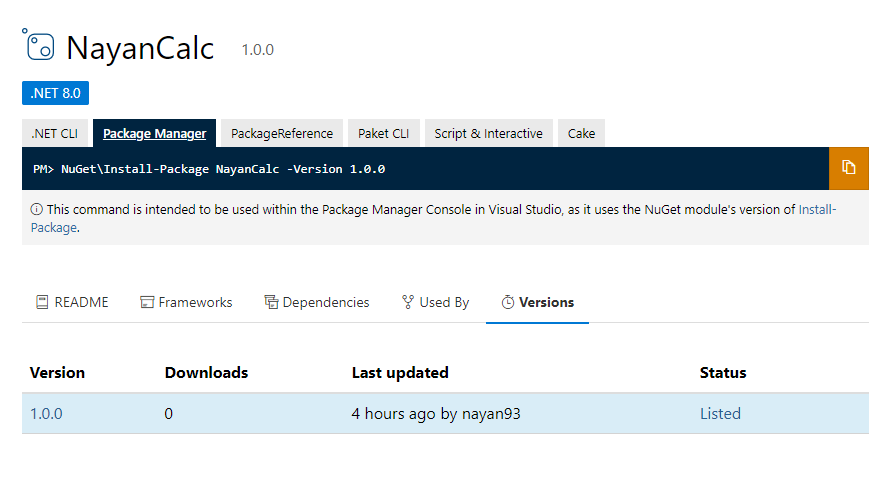
using Calc;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace NayanTest
{
public class test
{
public void MyMethod()
{
// Create an instance of the Calculator class
Calculator calculator = new Calculator();
// Now you can use the methods of the Calculator class
int result = calculator.Add(5, 10);
Console.WriteLine("Result of addition: " + result);
}
}
}
Create and publish a NuGet package using Visual Studio Read More »