Dependency Injection in C#
Dependency Injection (DI) is a type of IoC, it is a pattern where objects are not responsible for creating their own dependencies. Dependency injection is a way to remove hard-coded dependencies among objects, making it easier to replace an object’s dependencies, either for testing (using mock objects in unit test) or to change run-time behavior.
The result of this separation is a loosely coupled system where there is no rigid dependency between two concrete implementations.
Loose Coupling means two objects are independent and an object can use another object without being dependent on it. It is a design goal that seeks to reduce the inter- dependencies among components of a system with the goal of reducing the risk that changes in one component will require changes in any other component.
This pattern is a particular implementation where the consumer object receives its dependencies inside constructor, properties or arguments.
The dependency is an object (or a service object), which is passed as dependency to the consumer object (or a client application).
So generally we can say that, Dependency Injection is a pattern that makes objects loosely coupled instead of tightly coupled. Generally we create a concrete class object in the class we require the object and bind it in the dependent class but DI is a pattern where we create a concrete class object outside this high-level module or dependent class.
Types of Dependency Injection:
1. Constructor Injection:
- Constructor injection is the process of using the constructor to pass in the dependencies of a class.
- You should use constructor injection when your class has a dependency that the class requires in order to work properly.
- If your class cannot work without a dependency, then inject it via the constructor.
2. Property Injection:
- You should use property injection in case the dependency is truly optional
- Property Injection however causes Temporal Coupling and when writing Line of Business applications, your dependencies should never be optional: you should instead apply the Null Object pattern.
- Property injection is considered bad in 98% of all scenarios because it hides dependencies and there is no guarantee that the object will be injected when the class is created. (ref)
3. Method Injection:
- Method injection is useful in two scenarios:
- when the implementation of dependency will vary, and
- when the dependency needs to be renewed after each use.
In both cases, it’s up to the caller to decide what implementation to pass to the method
Role Of Unity
Unity container is an open source IoC container for .NET applications supported by Microsoft. It is a lightweight and extensible IoC container.
Unity Container Features:
- Simplified type-mapping registration for interface type or base type.
- Supports registration of an existing instance.
- Supports code-based registration as well as design time registration.
- Automatically injects registered type at runtime through a constructor, a property or a method.
- Supports deferred resolution.
- Supports nested containers.
Installation and Process of Unity Container
Here are steps to install unity library in visual studio:
- Create a project. It can be any type of project such as a class library, a console, a web, windows or any other C# or VB.NET project.
- After successfully project created , right click on project name in right side of solution explorer. And select Manage Nuget Packages.
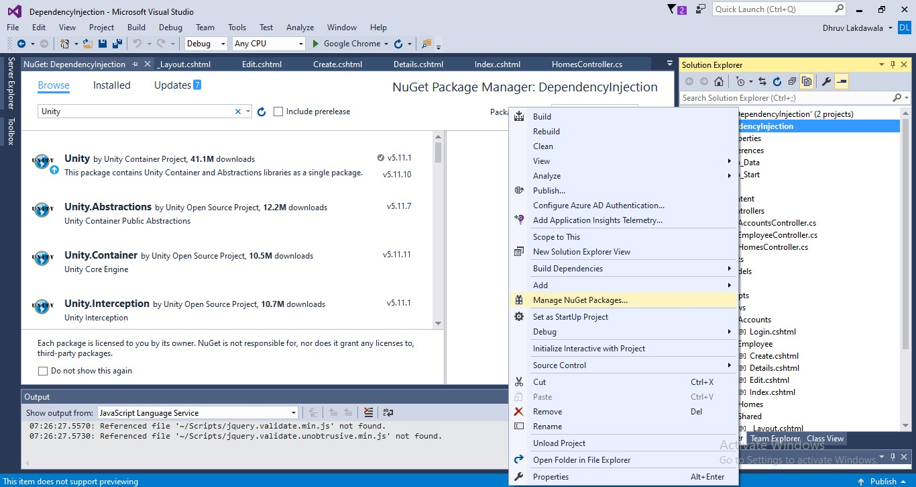
3. Nuget Package Dialog is open. In that dialog box goto browse tab and then search unity in search box.
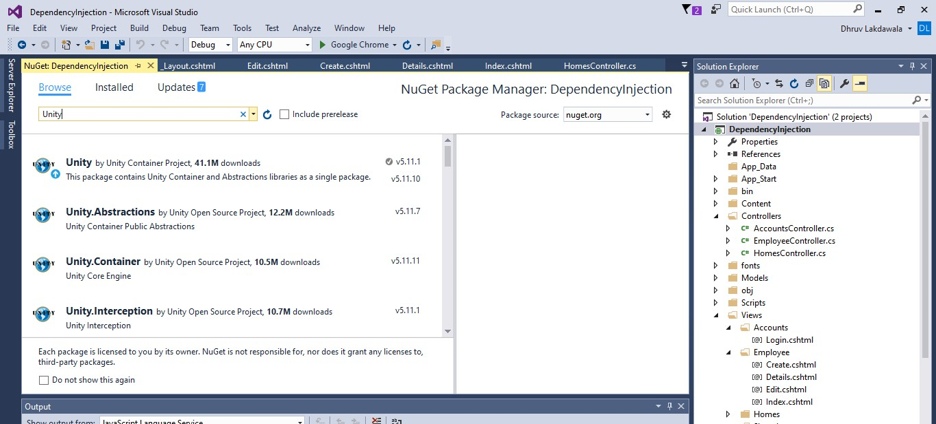
For Specific to MVC , you can also search “Unity.MVC”. There are so many unity library available for MVC. You can install as per your choice.
- After successfully unity library is installed one Config File named
“Unity.config” is generated in App_Start Folder.
– This file is heart of a dependency injection, using this file we can achieve dependency injection.
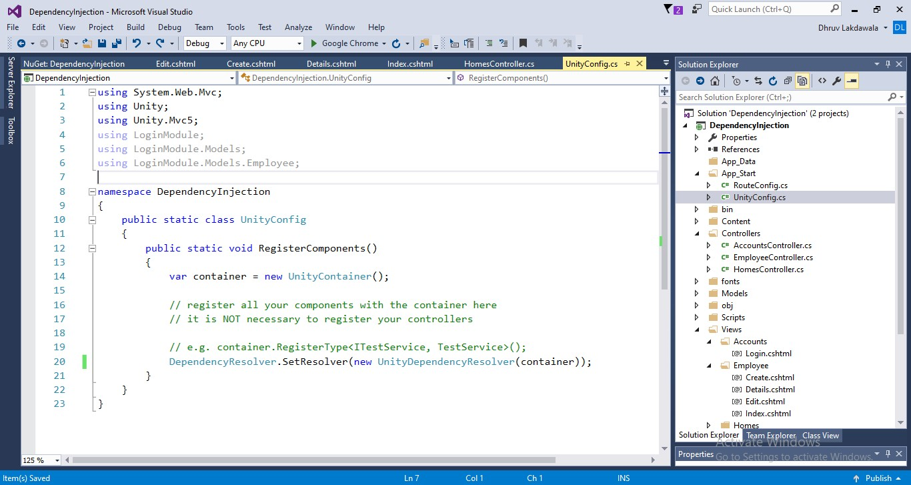
=> Register
Before Unity resolves the dependencies, we need to register the type-mapping with the container, so that it can create the correct object for the given type. Use the RegisterType() method to register a type mapping. Basically, it configures which class to instantiate for which interface or base class.
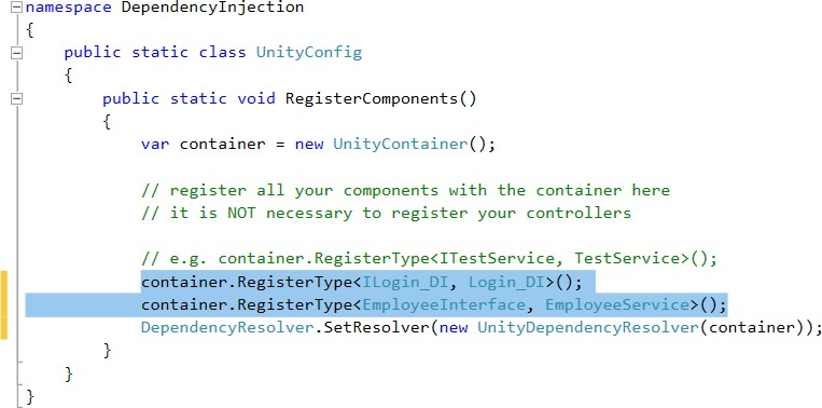
In above figure, if we want Unity container to create and supply an object of
the Login_DI class whenever it needs to supply a dependency of the ILogin_DI interface, then we first need to register.
Here, container.RegisterType<ILogin_DI, Login_DI>(); requests Unity to create an object of the Login_DI class and inject it through a constructor whenever you need to inject an object of ILogin_DI.
=>Resolving
Resolving the dependencies, can place at the controller side like this ILogin_DI objLogin = null;
//Constrctor Dependency
public AccountsController(ILogin_DI objILogin)
{
objLogin = objILogin;
}
Now, with the help of this objLogin Unity Container Object we can call the method of that class and resolve the dependency
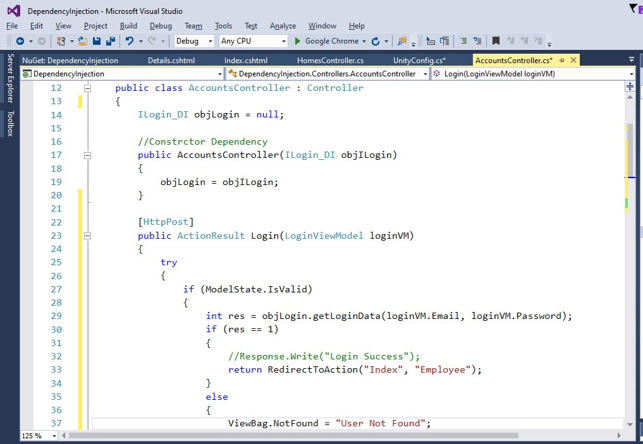
Dependency Injection in C# Read More »